Do you want to play the Rock Paper Scissors game with your EV3? If yes, there you go:
This project demonstrates how to react to EV3 Intelligent Brick button events, play built-in sound files, and make decisions using MicroPython. Please refer to my previous post LEGO EV3 MicroPython - Random Shapes on how to install MicroPython image for LEGO EV3.
Game Design
Rock paper scissors (also known by other permutations such as scissors paper rock, or ro-sham-bo) is a hand game usually played between two people, in which each player simultaneously forms one of three shapes with an outstretched hand. These shapes are “rock”, “paper”, and “scissors”.
The design of this game is straightforward:
- We need two variables
player
andcomputer
to hold player and EV3’s choices. Choices are in Python string type, i.e.,'rock'
,'paper'
, or'scissors'
. - Player uses EV3 Intelligent Brick buttons to make a selection (more on this in the later section). EV3 uses the built-in
urandom
module to get one of the choices randomly. - The general rules are:
- Rock crushes scissors.
- Paper covers rock.
- Scissors cuts paper.
Select a Winner
There are 3 tie cases, 3 winning cases, and 3 losing cases for each side.
Rock | Paper | Scissors | |
---|---|---|---|
Rock | Tie | Lose | Win |
Paper | Win | Tie | Lose |
Scissors | Lose | Win | Tie |
def select_winner(player, computer):
"""Return 'player' or 'computer' as the winner or None if tie"""
if player == computer:
return None
elif player == 'rock' and computer == 'scissors' \
or player == 'paper' and computer == 'rock' \
or player == 'scissors' and computer == 'paper':
return 'player'
else:
return 'computer'
Player Selection
To get the player input, we need a simple user interface. I have created one as shown below. Rock, paper, and scissors PNG images are loaded on program startup. A box is drawn around the image which indicates the current player selection.
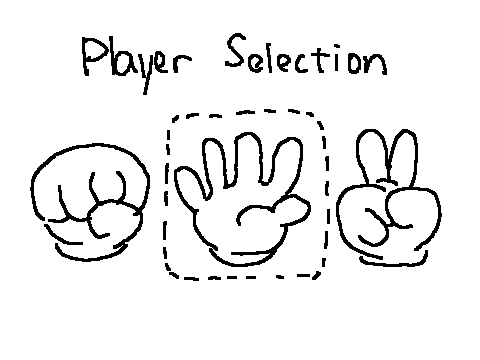
Player Selection Screen
Each image is scaled down to 48 pixels since the width of the EV3 Intelligent Brick screen is only 178 pixels. If you want to know more about image loading, I have a post LEGO EV3 MicroPython - I ❤️ Vancouver for this as well.
EV3 Intelligent Brick has 9 buttons on the its D-pad. buttons.pressed()
function will return a list of pressed buttons. I am using Button.LEFT
and Button.RIGHT
to make a selection and Button.CENTER
to confirm. player_selection()
function will return one of 'rock'
, 'paper'
, or 'scissors'
.
CHOICES = ['rock', 'paper', 'scissors']
def player_selection():
ev3.screen.draw_text(16, 8, 'Player Selection')
choices_len = len(CHOICES)
for index in range(choices_len):
x = 8 + index * (48 + 8) # 48 is the image width
y = 56
ev3.screen.draw_image(x, y, images[index])
index = 0
draw_box(index)
while True:
pressed_buttons = ev3.buttons.pressed()
if Button.CENTER in pressed_buttons:
break
elif Button.LEFT in pressed_buttons:
draw_box(index, True)
index = (index - 1) % choices_len
# turn negative index to positive
if index < 0:
index = choices_len - 1
draw_box(index)
ev3.speaker.say(CHOICES[index])
elif Button.RIGHT in pressed_buttons:
draw_box(index, True)
index = (index + 1) % choices_len
draw_box(index)
ev3.speaker.say(CHOICES[index])
wait(100)
return CHOICES[index]
The Main Loop
The main loop takes player input and randomize EV3’s selection. The winner is selected by select_winner(player, computer)
call. After the winner is selected, we clear the player selection screen and show the results screen.
EV3 MicroPython image ships with many built-in sound files in the media.ev3dev
module. We can play them by calling speaker.play_file(file_name)
.
while True:
# ev3 screen size is 178x128 pixels
ev3.screen.clear()
computer = urandom.choice(CHOICES)
player = player_selection()
# show result screen
ev3.screen.clear()
winner = select_winner(player, computer)
if winner == 'player':
ev3.speaker.say('You win!')
ev3.screen.draw_text(48, 96, 'You Win!')
ev3.speaker.play_file(SoundFile.CHEERING)
elif winner == 'computer':
ev3.speaker.say('You lose')
ev3.screen.draw_text(48, 96, 'You Lose')
ev3.speaker.play_file(SoundFile.BOO)
else:
ev3.speaker.say('Tie')
ev3.screen.draw_text(72, 96, 'Tie')
wait(3000)
EV3Brick Class Reference
buttons.pressed()
Checks which buttons are currently pressed.
screen.clear()
Clears the screen. All pixels on the screen will be set to Color.WHITE
.
speaker.play_file(file_name)
Plays a sound file.